Types Of Application :
1.Local Application –
Locally installed application , rich in look, performance is great.Disadvantage is – we cant access it remotely.
2.Remote Application –
Remotely accessed services or applications.Drawback is-they are technology specific Example : CORBA,Java RMI etc.
3.Web Services –
Loosely coupled client and server functionalities that run on particular network address.There are two types : SOAP -XML Based and Restful web services.
Introduction to Micro Services In Java
Micro Services Architecture
Very similar to Web services , micro services are functionalities that interact with each other over the network to perform some operation. Micro services is the extension of Web services ,and it is most latest architectural style to build distributed applications.
Micro services addresses single functionality or operation
Micro services are small , lightweight , use standard protocol
Micro services communicate with each other over network and every micro service built for single functionality :
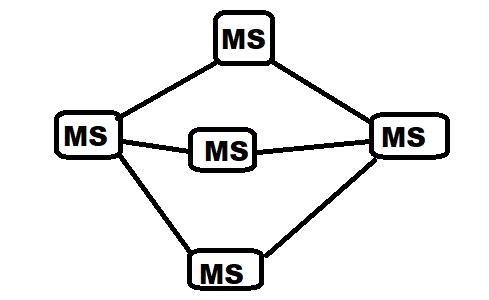
In Micro-services Architecture , we can develop different micro services using same or different technologies ,can have same database or different as below :

Advantages Of Micro services
Built for single functionality individually
Replacement of micro service is easy
Modifying one micro service will not affect on another
Scaling every functionality is possible
Micro service’s architecture is Horizontal Architecture
Micro services are small and address a single functionality, are robust , flexible and self-sufficient.
Do One Thing and Do It Well , is the thought behind micro services architecture.
In Monolithic application we use single technology for entire application , But ,in micro services architecture we have option to choose suitable technology for every individual micro service ,for example , micro service A we can develop using Java and micro service B we can develop using .NET and finally all micro services can act as a single application.
Disadvantages of Micro services :
complex to build at first.
Highest cost due to network calls.
Management of different micro services is complex.
Basic Example :
Sure! Let’s consider an example of two microservices in a Spring Boot-based microservices architecture: “Order Service” and “Payment Service.” The Order Service is responsible for handling order-related operations, while the Payment Service handles payment processing.
- Order Service:
The Order Service is responsible for managing orders and interacting with the Payment Service to process payments. It exposes RESTful APIs for creating orders, retrieving order details, and initiating the payment process. - Payment Service:
The Payment Service is responsible for processing payment transactions. It receives payment requests from the Order Service and interacts with external payment gateways or services to handle the payment processing. It exposes APIs for receiving payment requests and updating payment status.
Now, let’s understand the flow of interaction between these two microservices in depth:
Order Placement:
- A client sends a request to the Order Service API to create a new order.
- The Order Service receives the request and performs necessary validations and business logic.
- Once the order is validated and processed, the Order Service sends a request to the Payment Service API, passing the order details and the amount to be paid.
Payment Processing:
- The Payment Service receives the payment request from the Order Service.
- The Payment Service may perform additional validations on the payment request, such as checking the payment amount and verifying customer details.
- Upon successful validation, the Payment Service interacts with external payment gateways or services to process the payment transaction.
- Once the payment is processed, the Payment Service updates the payment status, indicating whether the payment was successful or not.
Order Status Update:
- After processing the payment, the Payment Service sends a response back to the Order Service, indicating the payment status.
- The Order Service receives the payment status response and updates the order status accordingly, such as marking the order as “Paid” or “Payment Failed.”
- The Order Service may perform additional actions based on the payment status, such as triggering notifications to the user or initiating further order processing.
It’s important to note that the communication between microservices can be implemented using various approaches, such as RESTful APIs, message queues (e.g., RabbitMQ, Apache Kafka), or gRPC. In this example, we assume RESTful APIs for simplicity.
To implement this scenario, you would typically develop the Order Service and Payment Service as separate Spring Boot applications. Each service would have its own controllers, services, and data repositories.
Furthermore, you would configure the communication between microservices using HTTP-based RESTful API calls. The Order Service would make an HTTP request to the Payment Service API, passing the necessary data in the request payload. The Payment Service would respond with the payment status, which the Order Service would then handle accordingly.
By following this architecture, you can achieve a decoupled and scalable microservices-based solution where each microservice handles its specific responsibility while collaborating with other microservices to accomplish end-to-end business processes.
Remember, this is a simplified example, and in real-world scenarios, additional considerations such as security, authentication, fault tolerance, and service discovery would need to be addressed.
Here’s an example code snippet for the Order Service and Payment Service in a Spring Boot microservices architecture.
This code demonstrates the interaction between the two services using RESTful APIs.
Order Service:
@RestController @RequestMapping("/orders") public class OrderController { @Autowired private RestTemplate restTemplate; // RestTemplate for making HTTP requests @PostMapping public ResponseEntitycreateOrder(@RequestBody Order order) { // Process order creation logic // Make an HTTP request to the Payment Service API String paymentServiceUrl = "http://payment-service/api/payments"; PaymentRequest paymentRequest = new PaymentRequest(order.getId(), order.getTotalAmount()); ResponseEntity paymentResponse = restTemplate.postForEntity(paymentServiceUrl, paymentRequest, String.class); // Process the payment response and update order status accordingly return ResponseEntity.ok("Order created successfully"); } // Other endpoints for retrieving order details, etc. }
Payment Service:
@RestController @RequestMapping("/api/payments") public class PaymentController { @PostMapping public ResponseEntityprocessPayment(@RequestBody PaymentRequest paymentRequest) { // Validate payment details // Process payment using external payment gateway or service // Update payment status return ResponseEntity.ok("Payment processed successfully"); } // Other endpoints for payment status retrieval, etc. }
Please note that this code is a simplified representation to showcase the interaction between the microservices. In a production environment, you would need to handle error scenarios, implement appropriate exception handling, add security measures, and configure service discovery if needed.
Additionally, you would need to set up the RestTemplate bean with appropriate configurations in the Order Service, and ensure that the necessary dependencies (e.g., Spring Web, RestTemplate) are included in the project’s build file (e.g., pom.xml for Maven).
Remember to adapt the code according to your specific requirements, including any additional validations, business logic, and error handling that may be necessary for your application.
Happy Learning.